This post contains the details about Labels support in Loops for break and continue construct.
Labelled Loops
In any programming paradigm a developer has to write some code dealing with loops. Loops are beneficial when we have to do some repetitive tasks. At-times most of the developers write the code of multiple loops, loops within a loop etc. In complex scenarios, there are situations in which a developer has to break out of the outer loop while iterating in an inner loop.
To expose this functionality, In Project Stratus(ColdFusion 2021) release, we have added the support of Labelled Loops using which a developer can provide a label before starting a loop and then use the constructs(break/continue) to break out or continue from the labelled loop from within an inner loop.
Labelled Loop Syntax:
* For Loop:
<OuterLabel> : for(){
<InnerLabel> : for(){
break OuterLabel;
continue OuterLabel;
}
}
* While Loop:
<OuterLabel> : while(){
<InnerLabel> : while(){
break OuterLabel;
continue OuterLabel;
}
}
Labels are the corresponding points which can be used as trace marks to break or continue within a loop. <Labels> will be used by corresponding break and continue statements to (break out)/(continue from) the corresponding loop at which the label is defined. In general if we write just break; or continue; statements it breaks out or continue from the closest loops from where the corresponding statement is executed.
Break and Continue Syntax:
break <label>; // where label is defined before the start of for/while loop
continue <label>; // where label is defined before the start of for/while loop
- continue statements
<
cfscript
>
xyz : for(i=110;i<115;i++){
abc : for(j=10;j<20;j++) {
writeDump(i);
writeOutput
(
"<br>"
);
if(j%3 == 0)
continue xyz;
writeDump(j);
}
writeDump(
"<br>"
);
}
</
cfscript
>
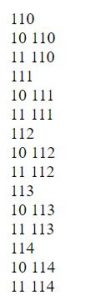
- Break Statement
<
cfscript
>
// breaking out using a label
x = 0;
WhileLabel: while (x < 10){
writeOutput
(
"x is #x#<br>"
);
switch (x){
case 1:
break;
case 3:
break WhileLabel;
}
x++;
writeOutput
(
"end of of loop<br>"
);
}
writeOutput
(
"After loop, x is #x#<br>"
);
</
cfscript
>
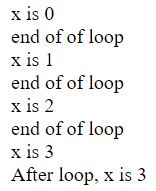
- Labelled For-in Loop
<
cfscript
>
myArray1 = [
"a"
,
"b"
,
"c"
,
"d"
,
"e"
];
xyz:for (currentIndex
in
myArray1)
{
if(#currentIndex#==
"d"
)
break xyz;
writeOutput
(currentIndex);
writeOutput
(
"<br>"
);
}
</
cfscript
>

<
cfloop
index=
"i"
from=
"1"
to=
"10"
label=
"xyz"
>
<
cfoutput
>#i#</
cfoutput
><br>
<
cfif
i Ge 5>
<cfcontinue xyz>
</
cfif
>
<
cfoutput
>#i#->#i#</
cfoutput
><br>
</
cfloop
>
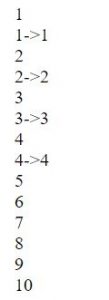
<
cftry
>
<
cfloop
index=
"i"
from=
"110"
to=
"114"
label=
"xyz"
>
<
cfloop
index=
"j"
from=
"10"
to=
"19"
label=
"abc"
>
<
cfoutput
>#i#<br></
cfoutput
>
<
cfif
j%3==0>
<cfcontinue xyz>
</
cfif
>
<
cfoutput
>#j#</
cfoutput
>
</
cfloop
>
</
cfloop
>
<
cfcatch
>
<
cfdump
var=
"#cfcatch#"
>
</
cfcatch
>
</
cftry
>
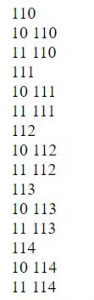
Note: I have given some examples here, but for the completeness, the labelled statements functionality is implemented in List/Array/Query and File based loops as well.
You must be logged in to post a comment.